Comment trouver la transposition d’une matrice dans plusieurs langues
La transposition d'une matrice est obtenue en intervertissant les lignes et les colonnes de la matrice d'origine. Dans cet article, vous apprendrez à trouver la transposition d'une matrice carrée et rectangulaire en utilisant C++, Python, JavaScript et C.
Énoncé du problème
On vous donne un tapis matriciel [][] . Vous devez trouver et imprimer la transposition de la matrice.
Exemples:
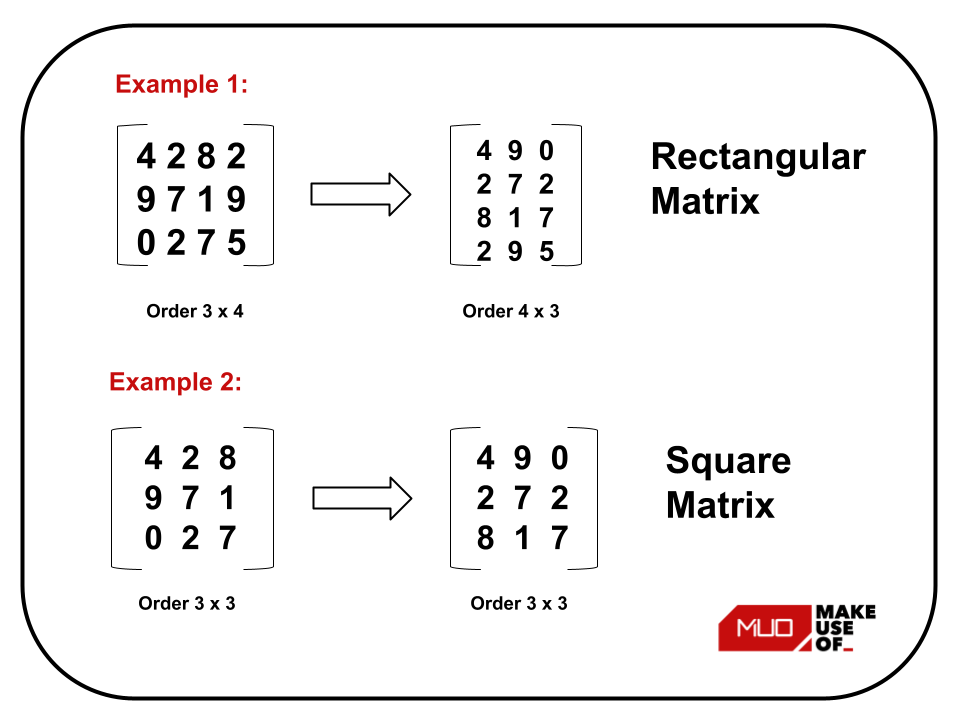
Comment trouver la transposition d'une matrice rectangulaire
- L'ordre de la transposition d'une matrice rectangulaire est opposé à celui de la matrice d'origine. Par exemple, si l'ordre de la matrice d'origine est 3 x 4, donc l'ordre de la transposition de cette matrice serait 4 x 3.
- Stockez chaque colonne de la matrice d'origine sous forme de lignes dans la matrice transposée, c'est-à-dire transposeMatrix[i][j] = mat[j][i].
Programme C++ pour trouver la transposition d'une matrice rectangulaire
Ci-dessous se trouve le programme C++ pour trouver la transposition d'une matrice rectangulaire :
// C++ program to find the transpose of a rectangular Matrix
#include <iostream>
using namespace std;
// The order of the initial matrix is 3 x 4
#define size1 3
#define size2 4
// Function to transpose a Matrix
void transposeMatrix(int mat[][size2], int transposeMatrix[][size1])
{
for (int i=0; i<size2; i++)
{
for (int j=0; j<size1; j++)
{
transposeMatrix[i][j] = mat[j][i];
}
}
}
// Driver Code
int main()
{
int mat[size1][size2] = { {4, 2, 8, 2},
{9, 7, 1, 9},
{0, 2, 7, 5} };
cout << "Initial Matrix:" << endl;
// Printing the initial Matrix
for (int i = 0; i < size1; i++)
{
for (int j = 0; j < size2; j++)
{
cout << mat[i][j] << " ";
}
cout << endl;
}
// Variable to store the transposed Matrix
// The dimensions of transposedMatrix are opposite to that of mat
int transposedMatrix[size2][size1];
transposeMatrix(mat, transposedMatrix);
cout << "Transposed Matrix:" << endl;
// Printing the transposed Matrix
for (int i = 0; i < size2; i++)
{
for (int j = 0; j < size1; j++)
{
cout << transposedMatrix[i][j] << " ";
}
cout << endl;
}
return 0;
}
Production:
Initial Matrix:
4 2 8 2
9 7 1 9
0 2 7 5
Transposed Matrix:
4 9 0
2 7 2
8 1 7
2 9 5
Programme Python pour trouver la transposition d'une matrice rectangulaire
Ci-dessous se trouve le programme Python pour trouver la transposition d'une matrice rectangulaire :
# Python program to find the transpose of a rectangular Matrix
# The order of the initial matrix is 3 x 4
size1 = 3
size2 = 4
# Function to transpose a Matrix
def transposeMatrix(mat, transposedMatrix):
for i in range(size2):
for j in range(size1):
transposedMatrix[i][j] = mat[j][i]
# Driver Code
mat = [ [4, 2, 8, 2],
[9, 7, 1, 9],
[0, 2, 7, 5] ]
print("Initial Matrix:")
# Printing the initial Matrix
for i in range(size1):
for j in range(size2):
print(mat[i][j], end=' ')
print()
# Variable to store the transposed Matrix
# The dimensions of transposedMatrix are opposite to that of mat
transposedMatrix = [[0 for x in range(size1)] for y in range(size2)]
transposeMatrix(mat, transposedMatrix)
print("Transposed Matrix:")
# Printing the transposed Matrix
for i in range(size2):
for j in range(size1):
print(transposedMatrix[i][j], end=' ')
print()
Production:
Initial Matrix:
4 2 8 2
9 7 1 9
0 2 7 5
Transposed Matrix:
4 9 0
2 7 2
8 1 7
2 9 5
Programme JavaScript pour trouver la transposition d'une matrice rectangulaire
Ci-dessous se trouve le programme JavaScript pour trouver la transposition d'une matrice rectangulaire :
// JavaScript program to find the transpose of a rectangular Matrix
// The order of the initial matrix is 3 x 4
var size1 = 3
var size2 = 4
// Function to transpose a Matrix
function transposeMatrix(mat, transposeMatrix) {
for (let i=0; i<size2; i++) {
for (let j=0; j<size1; j++) {
transposeMatrix[i][j] = mat[j][i];
}
}
}
// Driver Code
var mat = [ [4, 2, 8, 2],
[9, 7, 1, 9],
[0, 2, 7, 5] ];
document.write("Initial Matrix:" + "<br>");
// Printing the initial Matrix
for (let i = 0; i < size1; i++) {
for (let j = 0; j < size2; j++) {
document.write(mat[i][j] + " ");
}
document.write("<br>");
}
// Variable to store the transposed Matrix
// The dimensions of transposedMatrix are opposite to that of mat1
var transposedMatrix = new Array(size2);
for (let k = 0; k < size2; k++) {
transposedMatrix[k] = new Array(size1);
}
transposeMatrix(mat, transposedMatrix);
document.write("Transposed Matrix:" + "<br>");
// Printing the transposed Matrix
for (let i = 0; i < size2; i++) {
for (let j = 0; j < size1; j++) {
document.write(transposedMatrix[i][j] + " ");
}
document.write("<br>");
}
Production:
Initial Matrix:
4 2 8 2
9 7 1 9
0 2 7 5
Transposed Matrix:
4 9 0
2 7 2
8 1 7
2 9 5
C Programme pour trouver la transposition d'une matrice rectangulaire
Ci-dessous le programme C pour trouver la transposition d'une matrice rectangulaire :
// C program to find the transpose of a rectangular Matrix
#include <stdio.h>
// The order of the initial matrix is 3 x 4
#define size1 3
#define size2 4
// Function to transpose a Matrix
void transposeMatrix(int mat[][size2], int transposeMatrix[][size1])
{
for (int i=0; i<size2; i++)
{
for (int j=0; j<size1; j++)
{
transposeMatrix[i][j] = mat[j][i];
}
}
}
// Driver Code
int main()
{
int mat[size1][size2] = { {4, 2, 8, 2},
{9, 7, 1, 9},
{0, 2, 7, 5} };
printf("Initial Matrix: n");
// Printing the initial Matrix
for (int i = 0; i < size1; i++)
{
for (int j = 0; j < size2; j++)
{
printf("%d ", mat[i][j]);
}
printf("n");
}
// Variable to store the transposed Matrix
// The dimensions of transposedMatrix are opposite to that of mat1
int transposedMatrix[size2][size1];
transposeMatrix(mat, transposedMatrix);
printf("Transposed Matrix: n");
// Printing the transposed Matrix
for (int i = 0; i < size2; i++)
{
for (int j = 0; j < size1; j++)
{
printf("%d ", transposedMatrix[i][j]);
}
printf("n");
}
return 0;
}
Production:
Initial Matrix:
4 2 8 2
9 7 1 9
0 2 7 5
Transposed Matrix:
4 9 0
2 7 2
8 1 7
2 9 5
Comment trouver la transposition d'une matrice carrée
- L'ordre de transposition d'une matrice carrée est le même que celui de la matrice d'origine. Par exemple, si l'ordre de la matrice d'origine est de 3 x 3, l'ordre de transposition de cette matrice serait toujours de 3 x 3. Ainsi, déclarez une matrice avec le même ordre que celui de la matrice d'origine.
- Stockez chaque colonne de la matrice d'origine sous forme de lignes dans la matrice transposée, c'est-à-dire transposeMatrix[i][j] = mat[j][i].
Programme C++ pour trouver la transposition d'une matrice carrée
Ci-dessous le programme C++ pour trouver la transposition d'une matrice carrée :
// C++ program to find the transpose of a square matrix
#include <iostream>
using namespace std;
// The order of the matrix is 3 x 3
#define size 3
// Function to transpose a Matrix
void transposeMatrix(int mat[][size], int transposeMatrix[][size])
{
for (int i=0; i<size; i++)
{
for (int j=0; j<size; j++)
{
transposeMatrix[i][j] = mat[j][i];
}
}
}
int main()
{
int mat[size][size] = { {4, 2, 8},
{9, 7, 1},
{0, 2, 7} };
cout << "Initial Matrix:" << endl;
// Printing the initial Matrix
for (int i = 0; i < size; i++)
{
for (int j = 0; j < size; j++)
{
cout << mat[i][j] << " ";
}
cout << endl;
}
// Variable to store the transposed Matrix
int transposedMatrix[size][size];
transposeMatrix(mat, transposedMatrix);
cout << "Transposed Matrix:" << endl;
// Printing the transposed Matrix
for (int i = 0; i < size; i++)
{
for (int j = 0; j < size; j++)
{
cout << transposedMatrix[i][j] << " ";
}
cout << endl;
}
return 0;
}
Production:
Initial Matrix:
4 2 8
9 7 1
0 2 7
Transposed Matrix:
4 9 0
2 7 2
8 1 7
Programme Python pour trouver la transposition d'une matrice carrée
Ci-dessous se trouve le programme Python pour trouver la transposition d'une matrice carrée :
# Python program to find the transpose of a square Matrix
# The order of the initial matrix is 3 x 3
size = 3
# Function to transpose a Matrix
def transposeMatrix(mat, transposedMatrix):
for i in range(size):
for j in range(size):
transposedMatrix[i][j] = mat[j][i]
# Driver Code
mat = [ [4, 2, 8],
[9, 7, 1],
[0, 2, 7] ]
print("Initial Matrix:")
# Printing the initial Matrix
for i in range(size):
for j in range(size):
print(mat[i][j], end=' ')
print()
# Variable to store the transposed Matrix
transposedMatrix = [[0 for x in range(size)] for y in range(size)]
transposeMatrix(mat, transposedMatrix)
print("Transposed Matrix:")
# Printing the transposed Matrix
for i in range(size):
for j in range(size):
print(transposedMatrix[i][j], end=' ')
print()
Production:
Initial Matrix:
4 2 8
9 7 1
0 2 7
Transposed Matrix:
4 9 0
2 7 2
8 1 7
Programme JavaScript pour trouver la transposition d'une matrice carrée
Ci-dessous se trouve le programme JavaScript pour trouver la transposition d'une matrice carrée :
// JavaScript program to find the transpose of a square Matrix
// The order of the initial matrix is 3 x 3
var size = 3
// Function to transpose a Matrix
function transposeMatrix(mat, transposeMatrix) {
for (let i=0; i<size; i++) {
for (let j=0; j<size; j++) {
transposeMatrix[i][j] = mat[j][i];
}
}
}
// Driver Code
var mat = [ [4, 2, 8],
[9, 7, 1],
[0, 2, 7] ];
document.write("Initial Matrix:" + "<br>");
// Printing the initial Matrix
for (let i = 0; i < size; i++) {
for (let j = 0; j < size; j++) {
document.write(mat[i][j] + " ");
}
document.write("<br>");
}
// Variable to store the transposed Matrix
// The dimensions of transposedMatrix are opposite to that of mat1
var transposedMatrix = new Array(size);
for (let k = 0; k < size; k++) {
transposedMatrix[k] = new Array(size);
}
transposeMatrix(mat, transposedMatrix);
document.write("Transposed Matrix:" + "<br>");
// Printing the transposed Matrix
for (let i = 0; i < size; i++) {
for (let j = 0; j < size; j++) {
document.write(transposedMatrix[i][j] + " ");
}
document.write("<br>");
}
Production:
Initial Matrix:
4 2 8
9 7 1
0 2 7
Transposed Matrix:
4 9 0
2 7 2
8 1 7
Programme C pour trouver la transposition d'une matrice carrée
Ci-dessous le programme C pour trouver la transposition d'une matrice carrée :
// C program to find the transpose of a square Matrix
#include <stdio.h>
// The order of the initial matrix is 3 x 3
#define size 3
// Function to transpose a Matrix
void transposeMatrix(int mat[][size], int transposeMatrix[][size])
{
for (int i=0; i<size; i++)
{
for (int j=0; j<size; j++)
{
transposeMatrix[i][j] = mat[j][i];
}
}
}
// Driver Code
int main()
{
int mat[size][size] = { {4, 2, 8},
{9, 7, 1},
{0, 2, 7} };
printf("Initial Matrix: n");
// Printing the initial Matrix
for (int i = 0; i < size; i++)
{
for (int j = 0; j < size; j++)
{
printf("%d ", mat[i][j]);
}
printf("n");
}
// Variable to store the transposed Matrix
int transposedMatrix[size][size];
transposeMatrix(mat, transposedMatrix);
printf("Transposed Matrix: n");
// Printing the transposed Matrix
for (int i = 0; i < size; i++)
{
for (int j = 0; j < size; j++)
{
printf("%d ", transposedMatrix[i][j]);
}
printf("n");
}
return 0;
}
Production:
Initial Matrix:
4 2 8
9 7 1
0 2 7
Transposed Matrix:
4 9 0
2 7 2
8 1 7
Résoudre des problèmes de programmation de base basés sur des matrices
Une matrice est une grille utilisée pour stocker ou afficher des données dans un format structuré. Les matrices sont largement utilisées en programmation pour effectuer diverses opérations. Si vous cherchez à couvrir toutes les bases d'entretien de codage, vous devez savoir comment effectuer des opérations de base telles que l'addition, la soustraction, la multiplication, etc. sur des matrices.