Comment vérifier si deux matrices sont identiques avec la programmation
Deux matrices sont dites identiques si elles ont toutes les deux le même nombre de lignes, de colonnes et les mêmes éléments correspondants. Dans cet article, vous apprendrez à vérifier si deux matrices sont identiques à l'aide de Python, C++, JavaScript et C.
Énoncé du problème
On vous donne deux matrices mat1[][] et mat2[][] . Vous devez vérifier si les deux matrices sont identiques. Si les deux matrices sont identiques, imprimez "Oui, les matrices sont identiques". Et si les deux matrices ne sont pas identiques, imprimez "Non, les matrices ne sont pas identiques".
Exemples :
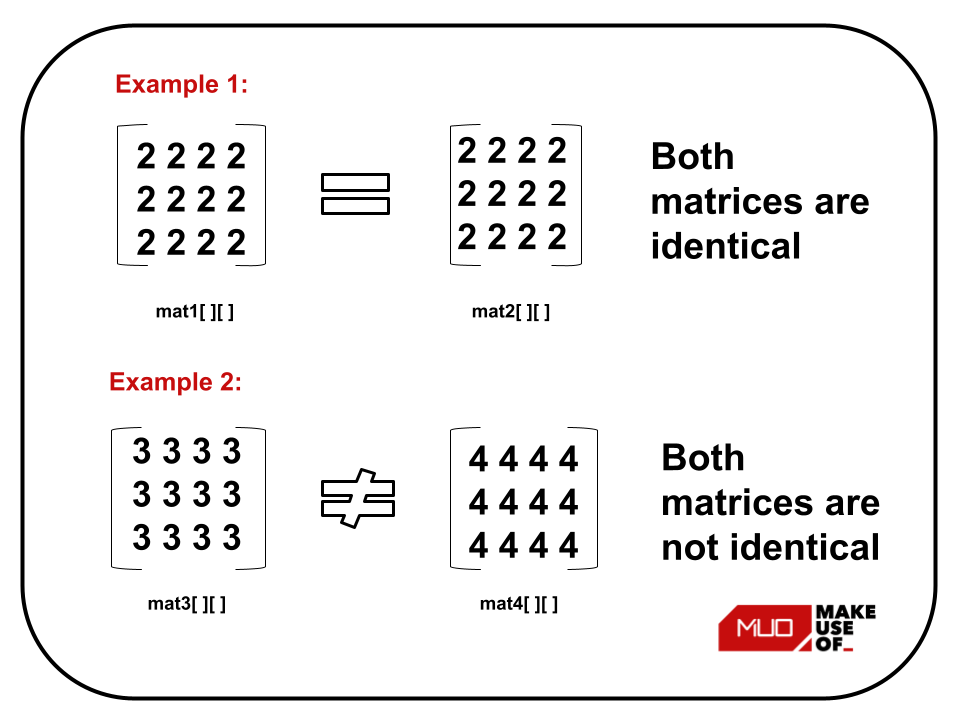
Condition pour que deux matrices soient identiques
Deux matrices sont dites identiques si et seulement si elles satisfont aux conditions suivantes :
- Les deux matrices ont le même nombre de lignes et de colonnes.
- Les deux matrices ont les mêmes éléments correspondants.
Approche pour vérifier si les deux matrices données sont identiques
Vous pouvez suivre l'approche ci-dessous pour vérifier si les deux matrices données sont identiques ou non :
- Exécutez une boucle imbriquée pour parcourir chaque élément des deux matrices.
- Si l'un des éléments correspondants des deux matrices n'est pas égal, renvoie false.
- Et si aucun élément correspondant n'est différent jusqu'à la fin de la boucle, retourne true.
Programme C++ pour vérifier si les deux matrices données sont identiques
Ci-dessous se trouve le programme C++ pour vérifier si les deux matrices données sont identiques ou non :
// C++ program to check if two matrices are identical
#include <bits/stdc++.h>
using namespace std;
// The order of the matrix is 3 x 4
#define size1 3
#define size2 4
// Function to check if two matrices are identical
bool isIdentical(int mat1[][size2], int mat2[][size2])
{
for (int i = 0; i < size1; i++)
{
for (int j = 0; j < size2; j++)
{
if (mat1[i][j] != mat2[i][j])
{
return false;
}
}
}
return true;
}
// Function to print a matrix
void printMatrix(int mat[][size2])
{
for (int i = 0; i < size1; i++)
{
for (int j = 0; j < size2; j++)
{
cout << mat[i][j] << " ";
}
cout << endl;
}
}
// Driver code
int main()
{
// 1st Matrix
int mat1[size1][size2] = { {2, 2, 2, 2},
{2, 2, 2, 2},
{2, 2, 2, 2} };
cout << "Matrix 1:" << endl;
printMatrix(mat1);
// 2nd Matrix
int mat2[size1][size2] = { {2, 2, 2, 2},
{2, 2, 2, 2},
{2, 2, 2, 2} };
cout << "Matrix 2:" << endl;
printMatrix(mat2);
if(isIdentical(mat1, mat2))
{
cout << "Yes, the matrices are identical" << endl;
}
else
{
cout << "No, the matrices are not identical" << endl;
}
// 3rd Matrix
int mat3[size1][size2] = { {3, 3, 3, 3},
{3, 3, 3, 3},
{3, 3, 3, 3} };
cout << "Matrix 3:" << endl;
printMatrix(mat3);
// 4th Matrix
int mat4[size1][size2] = { {4, 4, 4, 4},
{4, 4, 4, 4},
{4, 4, 4, 4} };
cout << "Matrix 4:" << endl;
printMatrix(mat4);
if(isIdentical(mat3, mat4))
{
cout << "Yes, the matrices are identical" << endl;
}
else
{
cout << "No, the matrices are not identical" << endl;
}
return 0;
}
Production:
Matrix 1:
2 2 2 2
2 2 2 2
2 2 2 2
Matrix 2:
2 2 2 2
2 2 2 2
2 2 2 2
Yes, the matrices are identical
Matrix 3:
3 3 3 3
3 3 3 3
3 3 3 3
Matrix 4:
4 4 4 4
4 4 4 4
4 4 4 4
No, the matrices are not identical
Programme Python pour vérifier si les deux matrices données sont identiques
Ci-dessous se trouve le programme Python pour vérifier si les deux matrices données sont identiques ou non :
# Python program to check if two matrices are identical
# The order of the matrix is 3 x 4
size1 = 3
size2 = 4
# Function to check if two matrices are identical
def isIdentical(mat1, mat2):
for i in range(size1):
for j in range(size2):
if (mat1[i][j] != mat2[i][j]):
return False
return True
# Function to print a matrix
def printMatrix(mat):
for i in range(size1):
for j in range(size2):
print(mat[i][j], end=' ')
print()
# Driver code
# 1st Matrix
mat1 = [ [2, 2, 2, 2],
[2, 2, 2, 2],
[2, 2, 2, 2] ]
print("Matrix 1:")
printMatrix(mat1)
# 2nd Matrix
mat2 = [ [2, 2, 2, 2],
[2, 2, 2, 2],
[2, 2, 2, 2] ]
print("Matrix 2:")
printMatrix(mat2)
if (isIdentical(mat1, mat2)):
print("Yes, the matrices are identical")
else:
print("No, the matrices are not identical")
# 3rd Matrix
mat3 = [ [3, 3, 3, 3],
[3, 3, 3, 3],
[3, 3, 3, 3] ]
print("Matrix 3:")
printMatrix(mat3)
# 4th Matrix
mat4 = [ [4, 4, 4, 4],
[4, 4, 4, 4],
[4, 4, 4, 4] ]
print("Matrix 4:")
printMatrix(mat4)
if (isIdentical(mat3, mat4)):
print("Yes, the matrices are identical")
else:
print("No, the matrices are not identical")
Production:
Matrix 1:
2 2 2 2
2 2 2 2
2 2 2 2
Matrix 2:
2 2 2 2
2 2 2 2
2 2 2 2
Yes, the matrices are identical
Matrix 3:
3 3 3 3
3 3 3 3
3 3 3 3
Matrix 4:
4 4 4 4
4 4 4 4
4 4 4 4
No, the matrices are not identical
Programme JavaScript pour vérifier si les deux matrices données sont identiques
Ci-dessous se trouve le programme JavaScript pour vérifier si les deux matrices données sont identiques ou non :
// JavaScript program to check if two matrices are identical
// The order of the matrix is 3 x 4
var size1 = 3;
var size2 = 4;
// Function to check if two matrices are identical
function isIdentical(mat1, mat2) {
for (let i = 0; i < size1; i++)
{
for (let j = 0; j < size2; j++)
{
if (mat1[i][j] != mat2[i][j])
{
return false;
}
}
}
return true;
}
// Function to print a matrix
function printMatrix(mat) {
for (let i = 0; i < size1; i++) {
for (let j = 0; j < size2; j++) {
document.write(mat[i][j] + " ");
}
document.write("<br>");
}
}
// Driver code
// 1st Matrix
var mat1 = [ [2, 2, 2, 2],
[2, 2, 2, 2],
[2, 2, 2, 2] ];
document.write("Matrix 1:" + "<br>");
printMatrix(mat1);
// 2nd Matrix
var mat2 = [ [2, 2, 2, 2],
[2, 2, 2, 2],
[2, 2, 2, 2] ];
document.write("Matrix 2:" + "<br>");
printMatrix(mat2);
if (isIdentical(mat1, mat2)) {
document.write("Yes, the matrices are identical" + "<br>");
} else{
document.write("No, the matrices are not identical" + "<br>");
}
// 3rd Matrix
var mat3 = [ [3, 3, 3, 3],
[3, 3, 3, 3],
[3, 3, 3, 3] ];
document.write("Matrix 3:" + "<br>");
printMatrix(mat3);
// 4th Matrix
var mat4 = [ [4, 4, 4, 4],
[4, 4, 4, 4],
[4, 4, 4, 4] ];
document.write("Matrix 4:" + "<br>");
printMatrix(mat4);
if (isIdentical(mat3, mat4)) {
document.write("Yes, the matrices are identical" + "<br>");
} else{
document.write("No, the matrices are not identical" + "<br>");
}
Production:
Matrix 1:
2 2 2 2
2 2 2 2
2 2 2 2
Matrix 2:
2 2 2 2
2 2 2 2
2 2 2 2
Yes, the matrices are identical
Matrix 3:
3 3 3 3
3 3 3 3
3 3 3 3
Matrix 4:
4 4 4 4
4 4 4 4
4 4 4 4
No, the matrices are not identical
C Programme pour vérifier si les deux matrices données sont identiques
Ci-dessous se trouve le programme C pour vérifier si les deux matrices données sont identiques ou non :
// C program to check if two matrices are identical
#include <stdio.h>
#include <stdbool.h>
// The order of the matrix is 3 x 4
#define size1 3
#define size2 4
// Function to check if two matrices are identical
bool isIdentical(int mat1[][size2], int mat2[][size2])
{
for (int i = 0; i < size1; i++)
{
for (int j = 0; j < size2; j++)
{
if (mat1[i][j] != mat2[i][j])
{
return false;
}
}
}
return true;
}
// Function to print a matrix
void printMatrix(int mat[][size2])
{
for (int i = 0; i < size1; i++)
{
for (int j = 0; j < size2; j++)
{
printf("%d ", mat[i][j]);
}
printf("n");
}
}
// Driver code
int main()
{
// 1st Matrix
int mat1[size1][size2] = { {2, 2, 2, 2},
{2, 2, 2, 2},
{2, 2, 2, 2} };
printf("Matrix 1:n");
printMatrix(mat1);
// 2nd Matrix
int mat2[size1][size2] = { {2, 2, 2, 2},
{2, 2, 2, 2},
{2, 2, 2, 2} };
printf("Matrix 2:n");
printMatrix(mat2);
if(isIdentical(mat1, mat2))
{
printf("Yes, the matrices are identical n");
}
else
{
printf("No, the matrices are not identical n");
}
// 3rd Matrix
int mat3[size1][size2] = { {3, 3, 3, 3},
{3, 3, 3, 3},
{3, 3, 3, 3} };
printf("Matrix 3: n");
printMatrix(mat3);
// 4th Matrix
int mat4[size1][size2] = { {4, 4, 4, 4},
{4, 4, 4, 4},
{4, 4, 4, 4} };
printf("Matrix 4: n");
printMatrix(mat4);
if(isIdentical(mat3, mat4))
{
printf("Yes, the matrices are identical n");
}
else
{
printf("No, the matrices are not identical n");
}
return 0;
}
Production:
Matrix 1:
2 2 2 2
2 2 2 2
2 2 2 2
Matrix 2:
2 2 2 2
2 2 2 2
2 2 2 2
Yes, the matrices are identical
Matrix 3:
3 3 3 3
3 3 3 3
3 3 3 3
Matrix 4:
4 4 4 4
4 4 4 4
4 4 4 4
No, the matrices are not identical
Apprendre un nouveau langage de programmation
L'informatique se développe à un rythme très rapide et la concurrence dans ce domaine est plus intense que jamais. Vous devez vous tenir au courant des dernières compétences et langages de programmation. Que vous soyez un programmeur débutant ou expérimenté, dans tous les cas, vous devriez apprendre certains des langages de programmation en fonction des exigences de l'industrie.